It’s advisable to keep source files for the assets (ex.: .ps, .max, .blend) near
the final ones you actually want Unity to import because it’s a lot easier to
keep things organized this way and work with Version Control Systems. Folks will
tell you to keep a mirror structure outside of assets folder, which is the exact
opposite.
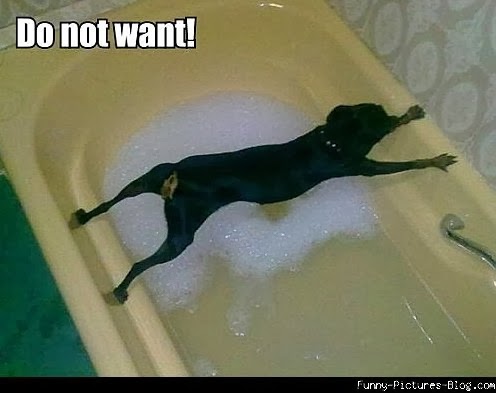
So here’s the trick: Unity doesn’t import files and folders that are hidden or
have the name starting with a dot. Naming files with a starting dot is better in
my opinion. Git for example doesn’t store the hidden attribute, and other VCSes
probably don’t as well.
You can do something like this:
.monkey.max <- source file, not imported
monkey.fbx <- imported normally
.monkey_diffuse.psd <- source file, not imported
monkey_diffuse.png <- imported normally
or
monkey.fbx <- imported normally
monkey_diffuse.png <- imported normally
.source <- entire folder not imported
.source/monkey.max
.source/monkey_diffuse.psd
The second structure can be better with many files. Windows explorer won’t let
you name a directory this way though (THANK YOU MICROSOFT!). Create or rename it
and write “.source.” (with final dot added), it will name it “.source” stripping
the final dot. Tested on Windows 7, don’t ask me why it’s like this.
Hopefully it will save you some headaches.